Обзор типов оконных приложений в C#. Знакомство со структурой проекта WPF/Avalonia. Компоновка. Image.
Взаимодействие кода C# и XAML
В MainWindow.xaml.cs
using System.Windows.Controls;
using System.Windows;
namespace WpfApp1
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void Button_Click(
object sender,
RoutedEventArgs e)
{
string text = textBox1.Text;
if (text != "")
{
MessageBox.Show(text);
}
}
}
}
В MainWindow.xaml
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid x:Name="grid1">
<TextBox
x:Name="textBox1"
Width="150"
Height="30"
VerticalAlignment="Top"
Margin="20" />
<Button
x:Name="button1"
Width="100"
Height="30"
Content="Кнопка"
Click="Button_Click" />
</Grid>
</Window>
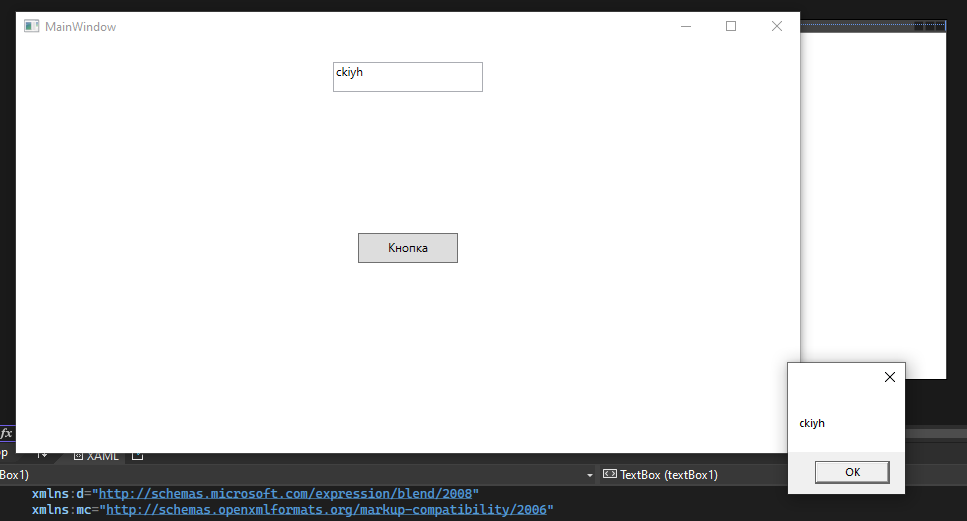
Пространства имен из C# в XAML
В MainWindow.xaml.cs
using System.Windows.Controls;
using System.Windows;
namespace WpfApp1
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
}
public class Phone
{
public string Name { get; set; }
public int Price { get; set; }
public override string ToString()
{
return $"Смартфон {this.Name}; цена: {this.Price}";
}
}
}
В MainWindow.xaml
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid x:Name="layoutGrid">
<Button
x:Name="phoneButton"
Width="250"
Height="40"
HorizontalAlignment="Center"
>
<Button.Content>
<local:Phone
Name="Lumia 950"
Price="700" />
</Button.Content>
</Button>
</Grid>
</Window>
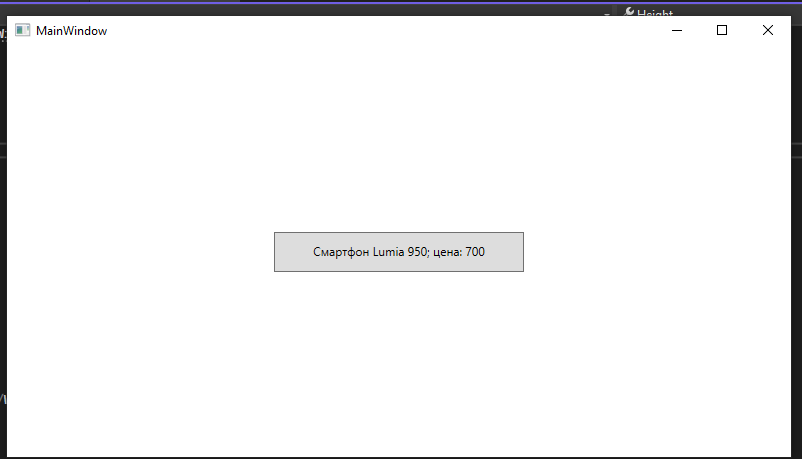
Grid (сетка)
В MainWindow.xaml
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid ShowGridLines="True">
<Grid.RowDefinitions>
<RowDefinition />
<RowDefinition />
<RowDefinition />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition />
<ColumnDefinition />
<ColumnDefinition />
</Grid.ColumnDefinitions>
<Button
Content="Строка 0 Столбец 0" />
<Button
Grid.Column="0"
Grid.Row="1"
Content="Объединение трех столбцов"
Grid.ColumnSpan="3" />
<Button
Grid.Column="2"
Grid.Row="2"
Content="Строка 2 Столбец 2" />
</Grid>
</Window>
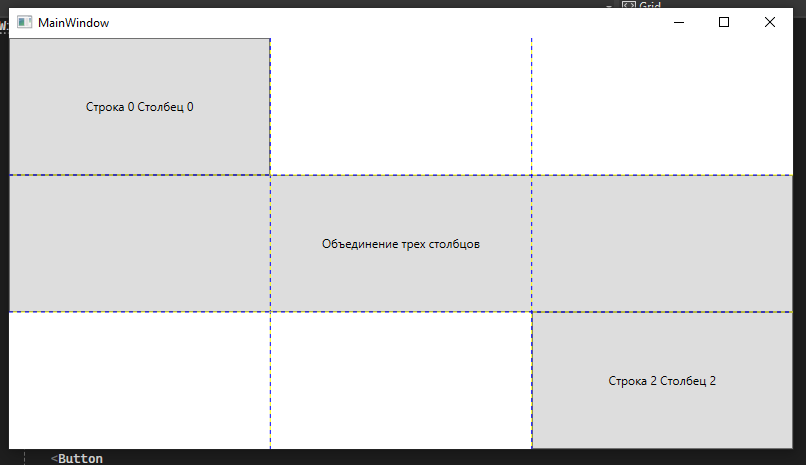
StackPanel
В MainWindow.xaml
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<StackPanel Orientation="Horizontal">
<Button
Background="Blue"
MinWidth="30"
Content="1" />
<Button
Background="White"
MinWidth="30"
Content="2" />
<Button
Background="Red"
MinWidth="30"
Content="3" />
</StackPanel>
</Window>
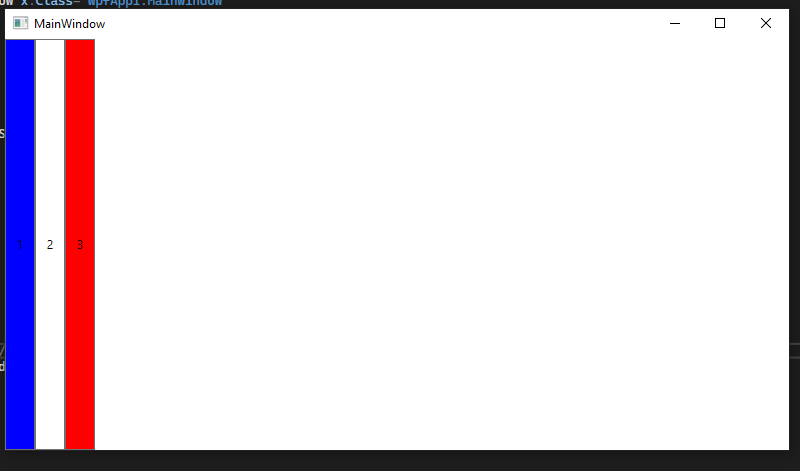
WrapPanel
В MainWindow.xaml
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<WrapPanel>
<Button
Background="AliceBlue"
Content="Кнопка 1" />
<Button
Background="Blue"
Content="Кнопка 2" />
<Button
Background="Aquamarine"
Content="Кнопка 3"
Height="30"/>
<Button
Background="DarkGreen"
Content="Кнопка 4"
Height="20"/>
<Button
Background="LightGreen"
Content="Кнопка 5"/>
<Button
Background="RosyBrown"
Content="Кнопка 6"
Width="80" />
<Button
Background="GhostWhite"
Content="Кнопка 7" />
</WrapPanel>
</Window>

<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<WrapPanel
ItemHeight="30"
ItemWidth="80"
Orientation="Horizontal"
>
<Button
Background="AliceBlue"
Content="1" />
<Button
Background="Blue"
Content="2" />
<Button
Background="Aquamarine"
Content="3"/>
<Button
Background="DarkGreen"
Content="4"/>
<Button
Background="LightGreen"
Content="5"/>
<Button
Background="AliceBlue"
Content="6" />
<Button
Background="Blue"
Content="7" />
</WrapPanel>
</Window>
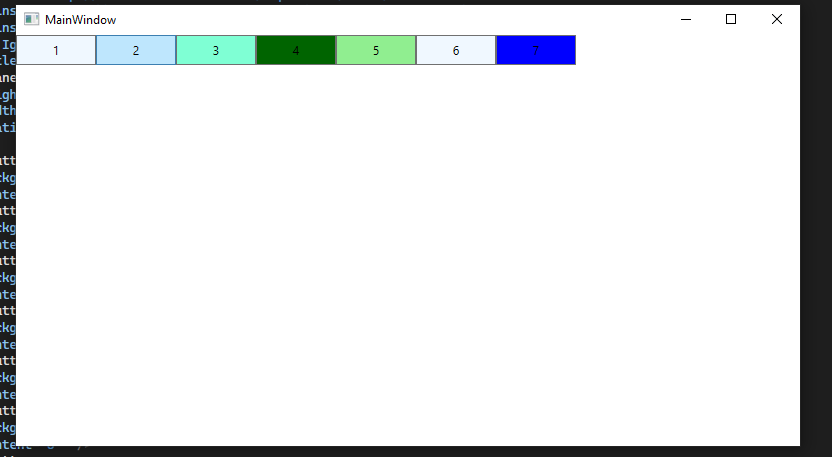